# Vue 系统课 -《艾鲜生》项目 API 接口文档
TIP
本接口文档旨在为《艾鲜生》项目的开发者提供详细、准确的 API 接口信息,以便其能够顺利地进行前后端的数据交互。
文档中包含了用户登录、注册接口,首页相关接口,店铺接口,订单接口,以及物流信息相关接口等关键功能点的详细说明。
- 用户注册接口,手机号注册、验证、短信验证码,用户注册
- 用户登录接口,验证码登录、Token 认证
- 搜索接口,banner 接口
- 菜单接口,秒杀商品接口、附近店铺推荐接口
- 店铺接口,店铺详情、商铺数据、商品详情
- 订单接口,生成订单、订单列表
- 物流信息相关接口,增删改查物流信息
# 一、注册接口
TIP
注册相关接口,包括:验证手机号是否注册、验证用户名是否被注册、获取用户手机号注册短信验证码、验证注册验证码是否有效、短信验证码注册用户
相关 API 接口根地址:https://api.icodingedu.cn
# 1、POST 验证手机号是否注册
TIP
- 接口 URL:
/api/register/phone/check
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 12687383488 | 是 | number |
- axios 请求示例
// 手机号是否注册
export function phoneIsExisted(phone: number) {
return axios.post<boolean, boolean>("/api/register/phone/check", {
phone,
});
}
- 响应数据示例
{
"code": 0,
"msg": "手机号未注册",
"data": false
}
{
"code": 0,
"msg": "手机号已注册",
"data": true
}
# 2、POST 验证用户名是否被注册
TIP
- 接口 URL:
/api/register/username/check
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
username (用户名) | admin123 | 是 | string |
- axios 请求示例
// 用户名是否注册
export function usernameIsExisted(username: string) {
return axios.post<boolean, boolean>("/api/register/username/check", {
username,
});
}
- 响应数据示例
{
"code": 0,
"msg": "用户名已注册",
"data": true
}
{
"code": 0,
"msg": "用户名未注册",
"data": false
}
# 3、POST 获取用户手机号注册短信验证码
TIP
- 接口 URL:
/api/register/code/send
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 13687383444 | 是 | number |
- axios 请求示例
interface ISmsSendCode {
code: string;
[prop: string]: string;
}
export function registerCodeSend(phone: number) {
return axios.post<ISmsSendCode, ISmsSendCode>("/api/register/code/send", {
phone,
});
}
- 响应数据示例--(成功)
{
"code": 0,
"msg": "短信发送成功",
"data": {
"Message": "OK",
"RequestId": "EF1EB9EE-2495-5BD4-B9DA-6DCDD3802D84",
"Code": "OK",
"BizId": "205002000408480018^0"
}
}
# 4、POST 验证注册验证码是否有效
TIP
- 接口 URL:
/api/register/code/check
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 12345677044 | 是 | number |
code | 123456 | 是 | string |
- axios 请求示例
interface ISmsCheckCode {
ACK: string;
}
// 验证验证码是否过期或无效
export function registerCodeCheck(phone: number, code: string) {
return axios.post<ISmsCheckCode, ISmsCheckCode>("/api/register/code/check", {
phone,
code,
});
}
- 响应数据示例
{
"code": 0,
"msg": "验证码正确,同时未过期",
"data": {
"ACK": "ok"
}
}
{
"code": 0,
"msg": "验证码不正确,或已过期",
"data": {
"ACK": "no"
}
}
# 5、POST 短信验证码注册用户
TIP
- 接口 URL:
/api/sms/register
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 12687383488 | 是 | number |
username (用户名) | admin1234 | 是 | string |
password(密码) | @Admin123 | 是 | string |
code(验证码) | 123456 | 是 | string |
- axios 请求示例
interface IUserInfo {
phone: number;
username: string;
password: string;
code: string;
}
export function smsRegistor({ phone, username, password, code }: IUserInfo) {
return axios.post("/api/sms/register", {
phone,
username,
password,
code,
});
}
- 响应数据示例
{
"code": 0,
"msg": "用户注册成功",
"data": {
"id": 1647738721573,
"phone": 13687383484,
"username": "admin1234",
"nickname": "ibc_xxxx",
"avatarUrl": "/images/avatar/avatar.png"
}
}
# 二、登录接口
TIP
登录相关接口,包括:发送登录短信验证码、验证登录验证码是否有效、短信验证码登录、Token 身份认证
# 1、POST 发送登录短信验证码
TIP
- 接口 URL:
/api/login/code/send
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 13687383485 | 是 | number |
- axios 请求示例
interface ISmsSendCode {
code: string;
[prop: string]: string;
}
export function smsLoginCodeSend(phone: number) {
return axios.post<ISmsSendCode, ISmsSendCode>("/api/login/code/send", {
phone,
});
}
- 响应数据示例
{
"code": 0,
"msg": "短信发送成功",
"data": {
"Message": "OK",
"RequestId": "0CB797ED-D068-568A-909D-D11D5BFA6DAE",
"Code": "OK",
"BizId": "681619497735655661^0"
}
}
# 2、POST 验证登录验证码是否有效
TIP
- 接口 URL:
/api/login/code/check
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 12687383488 | 是 | number |
code | 123456 | 是 | string |
- axios 请求示例
interface ISmsCheckCode {
ACK: string;
}
export function smsLoginCodeCheck(phone: number, code: string) {
return axios.post<ISmsCheckCode, ISmsCheckCode>("/api/login/code/check", {
phone,
code,
});
}
- 响应数据示例
{
"code": 0,
"msg": "验证码已过期",
"data": {
"ACK": "no"
}
}
{
"code": 0,
"msg": "验证码正确,同时未过期,可正常使用",
"data": {
"ACK": "ok"
}
}
# 3、POST 短信验证码登录
TIP
- 接口 URL:
/api/sms/login
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 |
---|---|---|---|
phone (手机号) | 12687383488 | 是 | number |
code ( 验证码 ) | 123456 | 是 | string |
- axios 请求示例
interface ILoginUserInfo {
userId: number;
phone?: number;
username?: string;
nickname: string;
avatarUrl: string;
}
interface IResponseLoginData {
userInfo: ILoginUserInfo;
token: string;
}
export function smsLogin(phone: number, code: string) {
return axios.post<IResponseLoginData, IResponseLoginData>("/api/sms/login", {
phone,
code,
});
}
- 响应数据示例
{
"code": 0,
"msg": "请求成功",
"data": {
"userInfo": {
"userId": 1700326685233,
"phone": 13687383486,
"username": "",
"nickname": "艾编程粉丝",
"avatarUrl": "/images/avatar/default.jpg"
},
"token": "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJ1c2VySWQiOjE3MDAzMjY2ODUyMzMsInBob25lIjoxMzY4NzM4MzQ4NiwidXNlcm5hbWUiOiIiLCJuaWNrbmFtZSI6IuiJvue8lueoi-eyieS4nSIsImF2YXRhclVybCI6Ii9pbWFnZXMvYXZhdGFyL2RlZmF1bHQuanBnIiwiaWF0IjoxNzAwNDA5OTQwLCJleHAiOjE3MDA0OTYzNDB9.Mrd8VrLNSjaqE4P0e8xF5jXcvM-BJ1TcZBOd-kMZ5Uw"
}
}
# 4、POST Token 身份认证
TIP
- 接口 URL:
/api/auth
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: Token 身份认证
- 注意: 发请求时,要在请求头的
authorization
字段中携带认证的 token
- axios 请求示例
export function auth() {
return axios.post < Boolean, Boolean > "/api/auth";
}
- 响应数据示例
{
"code": -1,
"msg": "身份认证失败",
"data": false
}
# 三、艾鲜生项目首页相关接口
TIP
艾鲜生项目首页相关接口,包括:获取搜索结果、获取首页 banner 信息、获取首页菜单数据、获取首页特价秒杀商品、获取首页附近推荐店铺
# 1、GET 获取搜索结果
TIP
- 接口 URL:
/api/search?keyword=水果&page=1&limit=10
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 身份认证
- Query 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
keyword | 水果 | 是 | string | 搜索的关键字 |
page | 1 | 否 | number | 当前页码 |
limit | 5 | 否 | number | 每页返回条数 |
补充说明:
当没有设置分页相关信息时,默认返回第 1 页前 10 条数据
- axios 请求示例
interface IDiscount {
type: number;
label: string;
content: { count: number; if: number }[];
}
interface IRedbag {
type: number;
count: number;
if: string;
label: string;
}
interface IService {
type: number;
value: string;
}
interface ISearchShopItem {
shopId: number;
avatarUrl: string;
shopName: string;
keyword: string[];
score: number;
monthlySales: number;
deliveryTime: string;
deliveryDistance: string;
deliveryStratingPrice: string;
deliveryStrategy: string;
deliveryTags: string;
comments: string[];
tops: string;
services: IService[];
redbags: IRedbag[];
discounts: IDiscount[];
announcement: string;
}
interface ISearchShopListData {
total: number;
data: ISearchShopItem[];
}
export function getSearchShopListData(
keyword: string,
page?: number,
limit?: number
) {
return axios.get<ISearchShopListData, ISearchShopListData>("/search", {
params: {
keyword,
page,
limit,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "请求成功",
"data": {
"total": 50,
"data": [
{
"shopId": 1,
"avatarUrl": "/images/logo/qg.png",
"shopName": "千xx丰水果(西安高简尚都店)",
"keyword": ["水果", "鱼肉", "蔬菜", "零食"],
"score": 4.8,
"monthlySales": 1000,
"deliveryTime": "46分钟",
"deliveryDistance": "4.3km",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "美团快递",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"tpye": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "支持自取"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客",
"label": "抢"
},
{
"type": 2,
"count": 6,
"if": "满39可用",
"label": "领"
},
{
"type": 2,
"count": 12,
"if": "满49可用",
"label": "领"
},
{
"type": 2,
"count": 20,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "千xx丰水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 2,
"avatarUrl": "/images/logo/bg.png",
"shopName": "百果xxx家(水果.果切.果捞.西安万科城店)",
"keyword": ["水果", "蔬菜", "饮料"],
"score": 3.8,
"monthlySales": 300,
"deliveryTime": "35分钟",
"deliveryDistance": "3.5km",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": [],
"tops": "长安区水果店回头率第9名",
"services": [
{
"tpye": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客立减",
"label": "领"
},
{
"type": 2,
"count": 15,
"if": "店铺通用满减 满79可用",
"label": "抢"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
}
]
}
],
"announcement": "百果xxx家,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 3,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 4,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 5,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 6,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 7,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 8,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 9,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 10,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
}
]
}
}
# 2、GET 获取首页 banner 信息
TIP
- 接口 URL:
/api/banner
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Query 参数 (无)
- axios 请求示例
interface IBannerItem {
id: number;
advUrl: string;
imageUrl: string;
}
type BannerList = IBannerItem[];
export function getBannerList() {
return axios.get<BannerList, BannerList>("/api/banner");
}
- 响应数据示例
{
"code": 0,
"msg": "请求成功",
"data": [
{
"id": 1,
"advUrl": "/shop/1",
"imageUrl": "/images/banner/banner1.png"
},
{
"id": 2,
"advUrl": "/goods-details/1/10003",
"imageUrl": "/images/banner/banner2.png"
},
{
"id": 3,
"advUrl": "/goods-details/1/10001",
"imageUrl": "/images/banner/banner3.png"
},
{
"id": 4,
"advUrl": "/my",
"imageUrl": "/images/banner/banner4.jpg"
},
{
"id": 5,
"advUrl": "/my",
"imageUrl": "/images/banner/banner5.jpg"
},
{
"id": 6,
"advUrl": "/my",
"imageUrl": "/images/banner/banner6.jpg"
}
]
}
# 3、GET 获取首页菜单数据
TIP
- 接口 URL:
/api/menu
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Query 参数 (无)
- axios 请求示例
interface MenuItem {
id: number;
title: string;
imgUrl: string;
href: string;
}
type MenuList = MenuItem[];
export function getMenuList() {
return axios.get<MenuList, MenuList>("/api/menu");
}
- 响应数据示例
{
"code": 0,
"msg": "请求成功",
"data": [
{
"id": 1,
"title": "美食",
"imgUrl": "/images/menu/delicacy.png",
"href": ""
},
{
"id": 2,
"title": "甜点饮品",
"imgUrl": "/images/menu/dessert.png",
"href": ""
},
{
"id": 3,
"title": "超市便利",
"imgUrl": "/images/menu/supermarket.png",
"href": ""
},
{
"id": 4,
"title": "蔬菜水果",
"imgUrl": "/images/menu/vegetable.png",
"href": ""
},
{
"id": 5,
"title": "买药看病",
"imgUrl": "/images/menu/medicine.png",
"href": ""
},
{
"id": 6,
"title": "晚餐",
"imgUrl": "/images/menu/dinner.png",
"href": ""
},
{
"id": 7,
"title": "拼好饭",
"imgUrl": "/images/menu/rice.png",
"href": ""
},
{
"id": 8,
"title": "津贴联盟",
"imgUrl": "/images/menu/moneybag.png",
"href": ""
},
{
"id": 9,
"title": "美食",
"imgUrl": "/images/menu/flower.png",
"href": ""
},
{
"id": 10,
"title": "跑腿",
"imgUrl": "/images/menu/run-errands.png",
"href": ""
}
]
}
# 4、GET 获取首页特价秒杀商品
TIP
- 接口 URL:
/api/seckill-timer
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Query 参数 (无)
- axios 请求示例
interface SeckillTimerGoods {
shopId: number;
goodId: number;
name: string;
imgUrl: string;
rating: string;
price: number;
oldPrice: number;
}
interface ISeckillTimer {
time: number;
goods: SeckillTimerGoods[];
}
export function getSeckillTimerGoods() {
return axios.get<ISeckillTimer, ISeckillTimer>("/api/seckill-timer");
}
- 响应数据示例
{
"code": 0,
"msg": "请求成功",
"data": {
"time": 172800000,
"goods": [
{
"shopId": 1,
"goodId": 10001,
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9
},
{
"shopId": 1,
"goodId": 10002,
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8
},
{
"shopId": 2,
"goodId": 20001,
"name": "招牌-雪梨绿果中(件)12粒1件",
"imgUrl": "/images/goods/type2-01.png",
"rating": "100%",
"price": 56.8,
"oldPrice": 76.8
},
{
"shopId": 1,
"goodId": 10005,
"name": "A级-阳光玫瑰青提(中)1份/480-500g",
"imgUrl": "/images/goods/type1-05.jpg",
"rating": "98%",
"price": 28.4,
"oldPrice": 31.5
},
{
"shopId": 1,
"goodId": 10006,
"name": "B级-洛川富士(大)1份/700-750g",
"imgUrl": "/images/goods/type1-06.jpg",
"rating": "98%",
"price": 13,
"oldPrice": 19.8
},
{
"shopId": 1,
"goodId": 10007,
"name": "【下单现剥】B级-红肉蜜柚1份/净果肉约350g",
"imgUrl": "/images/goods/type1-07.jpg",
"rating": "100%",
"price": 16.5,
"oldPrice": 18.8
},
{
"shopId": 1,
"goodId": 10008,
"name": "A级-玲珑小番茄(小)1份/230-250g",
"imgUrl": "/images/goods/type1-08.jpg",
"rating": "100%",
"price": 10.6,
"oldPrice": 11.5
}
]
}
}
# 5、GET 获取首页附近推荐店铺
TIP
- 接口 URL :
/api/recommend-shop?page=1&limit=10
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: 无需认证
- Query 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
page | 1 | 否 | number | 当前页码 |
limit | 5 | 否 | number | 每页返回条数 |
补充说明
当没有设置分页相关信息时,默认返回第 1 页前 10 条数据
- axios 请求示例
export interface IDiscount {
type: number;
label: string;
content: { count: number; if: number }[];
}
export interface IRedbag {
type: number;
count: number;
if: string;
label: string;
}
export interface IService {
type: number;
value: string;
}
export interface ISearchShopItem {
shopId: number;
avatarUrl: string;
shopName: string;
keyword: string[];
score: number;
monthlySales: number;
deliveryTime: string;
deliveryDistance: string;
deliveryStratingPrice: string;
deliveryStrategy: string;
deliveryTags: string;
comments: string[];
tops: string;
services: IService[];
redbags: IRedbag[];
discounts: IDiscount[];
announcement: string;
}
export interface ISearchShopListData {
total: number;
data: ISearchShopItem[];
}
export function getRecommendShop(page?: number, limit?: number) {
return axios.get<ISearchShopListData, ISearchShopListData>(
"/api/recommend-shop",
{
params: {
page,
limit,
},
}
);
}
- 响应数据示例
{
"code": 0,
"msg": "返回第1页的10条数据",
"data": {
"total": 50,
"data": [
{
"shopId": 1,
"avatarUrl": "/images/logo/qg.png",
"shopName": "千xx丰水果(西安高简尚都店)",
"keyword": ["水果", "鱼肉", "蔬菜", "零食"],
"score": 4.8,
"monthlySales": 1000,
"deliveryTime": "46分钟",
"deliveryDistance": "4.3km",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "美团快递",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"tpye": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "支持自取"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客",
"label": "抢"
},
{
"type": 2,
"count": 6,
"if": "满39可用",
"label": "领"
},
{
"type": 2,
"count": 12,
"if": "满49可用",
"label": "领"
},
{
"type": 2,
"count": 20,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "千xx丰水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 2,
"avatarUrl": "/images/logo/bg.png",
"shopName": "百果xxx家(水果.果切.果捞.西安万科城店)",
"keyword": ["水果", "蔬菜", "饮料"],
"score": 3.8,
"monthlySales": 300,
"deliveryTime": "35分钟",
"deliveryDistance": "3.5km",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": [],
"tops": "长安区水果店回头率第9名",
"services": [
{
"tpye": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客立减",
"label": "领"
},
{
"type": 2,
"count": 15,
"if": "店铺通用满减 满79可用",
"label": "抢"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
}
]
}
],
"announcement": "百果xxx家,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 3,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 4,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 5,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 6,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 7,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 8,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 9,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
},
{
"shopId": 10,
"avatarUrl": "/images/logo/qq.png",
"shopName": "百xx果园(西安金地未来地域)",
"keyword": ["水果", "鱼肉", "蔬菜"],
"score": 4.7,
"monthlySales": 1000,
"deliveryTime": "30分钟",
"deliveryDistance": "849m",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "蓝骑士",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"type": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "七天无理由退款"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客红包",
"label": "抢"
},
{
"type": 2,
"count": 15,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 3,
"if": 49
},
{
"count": 6,
"if": 59
},
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "xx水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
}
]
}
}
# 四、店铺接口
TIP
店铺相关接口,包括:获取店铺详情、获取店铺所有商品数据、获取商品详情
# 1、GET 获取店铺详情
TIP
- 接口 URL :
/api/shop/details?shopId=1
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Query 请求参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
shopId | 1 | 是 | number | 店铺 Id |
- axios 请求示例
interface IDiscount {
type: number;
label: string;
content: { count: number; if: number }[];
}
interface IRedbag {
type: number;
count: number;
if: string;
label: string;
}
interface IService {
type: number;
value: string;
}
export interface ISearchShopItem {
shopId: number;
avatarUrl: string;
shopName: string;
keyword: string[];
score: number;
monthlySales: number;
deliveryTime: string;
deliveryDistance: string;
deliveryStratingPrice: string;
deliveryStrategy: string;
deliveryTags: string;
comments: string[];
tops: string;
services: IService[];
redbags: IRedbag[];
discounts: IDiscount[];
announcement: string;
}
export function getShopDetailsByShopId(shopId: number) {
return axios.get<ISearchShopItem, ISearchShopItem>("/api/shop/details", {
params: {
shopId,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "返回信息成功",
"data": {
"shopId": 1,
"avatarUrl": "/images/logo/qg.png",
"shopName": "千xx丰水果(西安高简尚都店)",
"keyword": ["水果", "鱼肉", "蔬菜", "零食"],
"score": 4.8,
"monthlySales": 1000,
"deliveryTime": "46分钟",
"deliveryDistance": "4.3km",
"deliveryStratingPrice": "¥20.0",
"deliveryStrategy": "¥0",
"deliveryTags": "美团快递",
"comments": ["“红柚红心火龙果凤梨约600g三拼”"],
"tops": "雁塔区水果店销量第14名",
"services": [
{
"tpye": 1,
"value": "坏品包退"
},
{
"tpye": 2,
"value": "极速退款"
},
{
"tpye": 3,
"value": "支持自取"
},
{
"tpye": 4,
"value": "支持预订"
},
{
"tpye": 5,
"value": "开发票"
}
],
"redbags": [
{
"type": 1,
"count": 6,
"if": "新客",
"label": "抢"
},
{
"type": 2,
"count": 6,
"if": "满39可用",
"label": "领"
},
{
"type": 2,
"count": 12,
"if": "满49可用",
"label": "领"
},
{
"type": 2,
"count": 20,
"if": "满79可用",
"label": "领"
}
],
"discounts": [
{
"type": 1,
"label": "满减",
"content": [
{
"count": 8,
"if": 79
},
{
"count": 10,
"if": 99
}
]
}
],
"announcement": "千xx丰水果,活国亚运,杭州亚运会官方指定新鲜水果~诞生于1997年,全国水果连锁品牌。专注高品质鲜果,26年坚持全球优质产地直采,全国23大智慧冷链物流,让水果从枝头鲜到舌头,用水果让生活更美好。免费提供洗切服务,足不出户感受水果惬意~外卖轻松到家。"
}
}
# 2、GET 获取店铺所有商品数据
TIP
- 接口 URL:
/api/shop/goods?shopId=1
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Query 请求参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
shopId | 1 | 是 | number | 店铺 Id |
- axios 请求示例
export interface IGoods {
goodsId: string;
name: string;
imgUrl: string;
sellCount: number;
rating: string;
price: number;
oldPrice: number;
tips: string[];
discount: {
type: number;
value: number;
limitCount: number;
};
cartCount: number;
checked: boolean;
}
export interface IGoodsType {
typeId: number;
typename: string;
icon: string;
goods: IGoods[];
}
export interface IShopGoods {
shopId: number;
shopName: string;
data: IGoodsType[];
}
export function getShopGoodsByShopId(shopId: number) {
return axios.get<IShopGoods, IShopGoods>("/shop/goods", {
params: {
shopId,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "数据响应成功",
"data": {
"shopId": 1,
"shopName": "千xx丰水果(西安高简尚都店)",
"data": [
{
"typeId": 1,
"typename": "活动",
"icon": "",
"goods": [
{
"goodsId": "10001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 1,
"checked": true
},
{
"goodsId": "10002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "10003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "10004",
"name": "【爆品现切三拼350g】麒麟西瓜+密瓜+红心火龙果1份/净果肉350g",
"imgUrl": "/images/goods/type1-04.jpg",
"sellCount": 8,
"rating": "98%",
"price": 5.99,
"oldPrice": 20.9,
"tips": ["三拼"],
"discount": {
"type": 1,
"value": 2.87,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "10005",
"name": "A级-阳光玫瑰青提(中)1份/480-500g",
"imgUrl": "/images/goods/type1-05.jpg",
"sellCount": 10,
"rating": "98%",
"price": 28.4,
"oldPrice": 31.5,
"tips": ["青提", "国产中国云南省"],
"discount": {
"type": 1,
"value": 9.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "10006",
"name": "B级-洛川富士(大)1份/700-750g",
"imgUrl": "/images/goods/type1-06.jpg",
"sellCount": 11,
"rating": "98%",
"price": 13,
"oldPrice": 19.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 6.57,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "10007",
"name": "【下单现剥】B级-红肉蜜柚1份/净果肉约350g",
"imgUrl": "/images/goods/type1-07.jpg",
"sellCount": 18,
"rating": "100%",
"price": 16.5,
"oldPrice": 18.8,
"tips": ["单拼", "柚子切片"],
"discount": {
"type": 1,
"value": 8.78,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "10008",
"name": "A级-玲珑小番茄(小)1份/230-250g",
"imgUrl": "/images/goods/type1-08.jpg",
"sellCount": 28,
"rating": "100%",
"price": 10.6,
"oldPrice": 11.5,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 9.22,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 2,
"typename": "中秋礼盒",
"icon": "",
"goods": [
{
"goodsId": "20001",
"name": "招牌-猕宗绿果中(件)12粒1件",
"imgUrl": "/images/goods/type2-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 56.8,
"oldPrice": 76.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 1,
"checked": true
},
{
"goodsId": "20002",
"name": "礼盒金玉满堂礼盒了1份",
"imgUrl": "/images/goods/type2-01.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "20003",
"name": "【果篮】佳果芬芳果篮1份",
"imgUrl": "/images/goods/type2-01.jpg",
"sellCount": 65,
"rating": "98%",
"price": 199,
"oldPrice": 222,
"tips": ["包装精美", "箱装", "国产中国"],
"discount": {
"type": 1,
"value": 8.94,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 3,
"typename": "网红系列",
"icon": "",
"goods": [
{
"goodsId": "30001",
"name": "【网红小吃】乌梅菠萝1份/净果肉250g",
"imgUrl": "/images/goods/type3-01.jpg",
"sellCount": 6,
"rating": "100%",
"price": 27.7,
"oldPrice": 4.9,
"tips": ["单拼", "乌梅菠萝", "切块", "鲜果现切"],
"discount": {
"type": 1,
"value": 8.99,
"limitCount": 1
},
"cartCount": 1,
"checked": true
},
{
"goodsId": "30002",
"name": "【网红小吃】乌梅菠萝+麒麟西瓜1份/净果肉400g",
"imgUrl": "/images/goods/type3-01.jpg",
"sellCount": 14,
"rating": "100%",
"price": 33.9,
"oldPrice": 36.9,
"tips": ["双拼", "菠萝", "凤梨切片"],
"discount": {
"type": 1,
"value": 9.19,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "30003",
"name": "【网红小吃】DIY水果捞-下午茶-90分酸奶+香蕉1份",
"imgUrl": "/images/goods/type3-01.jpg",
"sellCount": 65,
"rating": "98%",
"price": 13.3,
"oldPrice": 14.5,
"tips": ["混合果捞"],
"discount": {
"type": 1,
"value": 9.17,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 4,
"typename": "单拼洗切",
"icon": "",
"goods": [
{
"goodsId": "40001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type4-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "40002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type4-01.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "40003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type4-01.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 5,
"typename": "冰镇现切",
"icon": "",
"goods": [
{
"goodsId": "50001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type5-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "50002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type5-01.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "50003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type5-01.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 6,
"typename": "多拼团餐",
"icon": "",
"goods": [
{
"goodsId": "60001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type6-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "60002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type6-01.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "60003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type6-01.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 7,
"typename": "瓜果飘香",
"icon": "",
"goods": [
{
"goodsId": "70001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "70002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "70003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 8,
"typename": "热带水果",
"icon": "",
"goods": [
{
"goodsId": "80001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "80002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "80003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 9,
"typename": "浆果葡提",
"icon": "",
"goods": [
{
"goodsId": "90001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "90002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "90003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 10,
"typename": "柑橘橙柚",
"icon": "",
"goods": [
{
"goodsId": "100001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "100002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "100003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 11,
"typename": "冰冻水果",
"icon": "",
"goods": [
{
"goodsId": "110001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "110002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "110003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 12,
"typename": "葡萄西梅",
"icon": "",
"goods": [
{
"goodsId": "120001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "120002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "120003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
},
{
"typeId": 13,
"typename": "双拼水果",
"icon": "",
"goods": [
{
"goodsId": "130001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "130002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
},
{
"goodsId": "130003",
"name": "【现切200g】B级-麒麟西瓜(中)1份/纯果肉200g",
"imgUrl": "/images/goods/type1-03.jpg",
"sellCount": 65,
"rating": "98%",
"price": 0.01,
"oldPrice": 5.5,
"tips": ["双拼", "水果混合果切", "果拼"],
"discount": {
"type": 1,
"value": 0.02,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
]
}
]
}
}
# 3、GET 获取商品详情
TIP
- 接口 URL:
/api/goods/details?shopId=1&goodsId=10002
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Query 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
shopId | 1 | 是 | number | 商铺 id |
goodsId | 10001 | 是 | number | 商品 id |
- axios 请求示例
interface IGoods {
goodsId: string;
name: string;
imgUrl: string;
sellCount: number;
rating: string;
price: number;
oldPrice: number;
tips: string[];
discount: {
type: number;
value: number;
limitCount: number;
};
cartCount: number;
checked: boolean;
}
interface IGoodsDetails {
shopId: number;
shopName: string;
goods: IGoods;
}
export function getGoodsDetailsById(shopId: number, goodsId: string) {
return axios.get<IGoodsDetails, IGoodsDetails>("/api/goods/details", {
params: {
shopId,
goodsId,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "成功返回对应商品数据",
"data": {
"shopId": "1",
"shopName": "千xx丰水果(西安高简尚都店)",
"goods": {
"goodsId": "10002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 0,
"checked": false
}
}
}
# 五、订单接口
TIP
订单相关接口,包括:生成用户订单、获取用户订单列表
# 1、POST 生成用户订单
TIP
- 接口 URL:
/api/generate/order
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
addressId | 1 | 是 | number | 收货地址 Id |
shopId | 11 | 是 | number | 店铺 Id |
shopName | 千 xxx 水果 | 是 | string | 店铺名称 |
isCanceled | true | 是 | boolean | 订单状态(取消或已完成) |
goodList | 是 | array | 订单对应商品列表 |
- axios 请求示例
interface IGoods {
goodsId: string;
name: string;
imgUrl: string;
sellCount: number;
rating: string;
price: number;
oldPrice: number;
tips: string[];
discount: {
type: number;
value: number;
limitCount: number;
};
cartCount: number;
checked: boolean;
}
interface IShopingCartInfos {
shopName: string;
goodsList: IGoods[];
}
export interface IOrderItem extends IShopingCartInfos {
userId: number;
orderId: number;
shopId: number;
addressId: number;
isCanceled: boolean;
}
export function generateOrder(orderItem: IOrderItem | IOrderItem[]) {
return axios.post<IOrderItem, IOrderItem>("/api/generate/order", orderItem);
}
- 响应数据示例
{
"code": 0,
"msg": "订单生成成功",
"data": {
"orderId": 1700467064327,
"userId": 1700326685233,
"shopId": 1,
"shopName": "千xx丰水果(西安高简尚都店)",
"addressId": 1700466822630,
"goodsList": [
{
"goodsId": "10002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 1,
"checked": true
}
],
"isCanceled": false
}
}
# 2、GET 获取用户订单列表
TIP
- 接口 URL:
/api/order/list
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Query 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
- axios 请求示例
interface IGoods {
goodsId: string;
name: string;
imgUrl: string;
sellCount: number;
rating: string;
price: number;
oldPrice: number;
tips: string[];
discount: {
type: number;
value: number;
limitCount: number;
};
cartCount: number;
checked: boolean;
}
interface IShopingCartInfos {
shopName: string;
goodsList: IGoods[];
}
export interface IOrderItem extends IShopingCartInfos {
userId: number;
orderId: number;
shopId: number;
addressId: number;
isCanceled: boolean;
}
export function getOrderList(userId: number) {
return axios.get<IOrderItem[], IOrderItem[]>("/order/list", {
params: {
userId,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "返回所有订单列表数据!",
"data": [
{
"orderId": 1700467064327,
"userId": 1700326685233,
"shopId": 1,
"shopName": "千xx丰水果(西安高简尚都店)",
"addressId": 1700466822630,
"goodsList": [
{
"goodsId": "10002",
"name": "B级-黄柠檬中个(1)个",
"imgUrl": "/images/goods/type1-02.jpg",
"sellCount": 9,
"rating": "100%",
"price": 0.01,
"oldPrice": 3.8,
"tips": ["国产"],
"discount": {
"type": 1,
"value": 0.03,
"limitCount": 1
},
"cartCount": 1,
"checked": true
}
],
"isCanceled": false
},
{
"orderId": 1700466831317,
"userId": 1700326685233,
"shopId": 1,
"shopName": "千xx丰水果(西安高简尚都店)",
"addressId": 1700466824631,
"goodsList": [
{
"goodsId": "10001",
"name": "B级-进口香蕉2根/300-350g",
"imgUrl": "/images/goods/type1-01.jpg",
"sellCount": 27,
"rating": "100%",
"price": 0.99,
"oldPrice": 4.9,
"tips": ["香甜软糯", "袋装", "国产"],
"discount": {
"type": 1,
"value": 2.02,
"limitCount": 1
},
"cartCount": 1,
"checked": true
}
],
"isCanceled": false
}
]
}
# 六、收货地址接口
TIP
物流信息相关接口,包括:获取收货地址、获取默认收货地址、新增收货地址、更新收货地址、删除单个收货地址
# 1、GET 获取收货地址
TIP
- 接口 URL:
/api/address
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Query 请求参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
addressId | 1 | 否 | number | 收货地址 id |
注:
- 只传
userId
获取当前用户的所有收获地址 - 如果
userId
和addressId
都传,则获取当前用户对应的某个地址
- axios 请求示例
type IAddress = IAddressItem | IAddressItem[];
interface IAddressItem {
userId: number;
addressId?: number;
area: string;
areaCode: string;
detailsAddress: string;
receiver: string;
phone: string;
isDefault: boolean;
}
export function getAddress(userId: number, addressId?: number) {
return axios.get<IAddress, IAddress>("/address", {
params: {
userId,
addressId,
},
});
}
响应数据示例
- 单个收获地址
{ "code": 0, "msg": "成功返回单个地址", "data": { "addressId": 1700466822630, "userId": 1700326685233, "receiver": "清心", "area": "辽宁省/沈阳市/和平区", "areaCode": "210102", "detailsAddress": "幸福路电子城街道银科大厦1栋5楼1206", "phone": "1367896789", "isDefault": false } }
- 当前用户所有收获地址
{ "code": 0, "msg": "成功返回所有收货地址信息", "data": [ { "addressId": 1700469462278, "userId": 1700326685233, "receiver": "清心", "area": "河北省/石家庄市/长安区", "areaCode": "130102", "detailsAddress": "快乐家园1栋2单", "phone": "18789432634", "isDefault": true }, { "addressId": 1700466822630, "userId": 1700326685233, "receiver": "清心", "area": "辽宁省/沈阳市/和平区", "areaCode": "210102", "detailsAddress": "幸福路电子城街道银科大厦1栋5楼1206", "phone": "1367896789", "isDefault": false } ] }
# 2、GET 获取默认收货地址
TIP
- 接口 URL:
/api/address/default
- 请求方式:GET
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Query 请求参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
- axios 请求示例
type IAddress = IAddressItem | IAddressItem[];
interface IAddressItem {
userId: number;
addressId?: number;
area: string;
areaCode: string;
detailsAddress: string;
receiver: string;
phone: string;
isDefault: boolean;
}
export function getDefaultAddress(userId: number) {
return axios.get<IAddressItem, IAddressItem>("/address/default", {
params: {
userId,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "成功返回默认地址",
"data": {
"addressId": 1700469462278,
"userId": 1700326685233,
"receiver": "清心",
"area": "河北省/石家庄市/长安区",
"areaCode": "130102",
"detailsAddress": "快乐家园1栋2单",
"phone": "18789432634",
"isDefault": true
}
}
# 3、POST 新增收货地址
TIP
- 接口 URL :
/api/address
- 请求方式:POST
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
receiver | 清心 | 是 | string | 收件人 |
area | 陕西省西安市长安区 | 是 | string | 省市区信息 |
areaCode | 省市区(区编码) | 是 | string | 区编码 |
detailsAddress | 电子城街道科技园 1 栋 5 楼 1206 | 是 | string | 详细地址 |
phone | 12332222221 | 是 | string | 收件人电话 |
isDefault | true | 是 | Boolean | 是否为默认地址 |
- axios 请求示例
export function addAddress(address: IAddressItem) {
return axios.post("/address", address);
}
- 响应数据示例
{
"code": 0,
"msg": "地址添加成功!",
"data": {
"addressId": 1700470223151,
"userId": 1700326685233,
"receiver": "清心",
"area": "上海市/上海市/黄浦区",
"areaCode": "310101",
"detailsAddress": "天桥家园2栋1单元202",
"phone": "12332222221",
"isDefault": false
}
}
# 4、PUT 更新收货地址
TIP
- 接口 URL:
/api/address
- 请求方式:PUT
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Body 参数
参数名 | 参数示例值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
addressId | 1 | 是 | number | 收货地址 id |
receiverName | 清心 | 是 | string | 收件人 |
area | 陕西省西安市长安区 | 是 | string | 省市区信息 |
houseNumber | 电子城街道科技园 1 栋 5 楼 1206 | 是 | string | 详细地址 |
tel | 12332222221 | 是 | string | 收件人电话 |
isDefault | true | 是 | Boolean | 是否为默认地址 |
- axios 请求示例
export function updateAddress(address: IAddressItem) {
return axios.put<IAddressItem, IAddressItem>("/address", address);
}
- 响应数据示例
{
"code": 0,
"msg": "更新成功",
"data": {
"addressId": 1700469462278,
"userId": 1700326685233,
"receiver": "清心",
"area": "河北省/石家庄市/长安区",
"areaCode": "130102",
"detailsAddress": "快乐家园1栋2单",
"phone": "18789432634",
"isDefault": false
}
}
# 5、DELETE 删除单个收货地址
TIP
- 接口 URL:
/api/address
- 请求方式:DELETE
- 响应数据类型:
Content-Type : application/json; charset=utf-8
- 认证方式: token 认证
- Body 参数
参数名 | 参数值 | 是否必填 | 参数类型 | 描述说明 |
---|---|---|---|---|
userId | 1700326685233 | 是 | number | 用户 Id |
addressId | 1 | 是 | number | 收货地址 Id |
- axios 请求示例
type IAddress = IAddressItem | IAddressItem[];
interface IAddressItem {
userId: number;
addressId?: number;
area: string;
areaCode: string;
detailsAddress: string;
receiver: string;
phone: string;
isDefault: boolean;
}
export function deleteAddress(userId: number, addressId: number) {
return axios.delete<IAddressItem, IAddressItem>("/address", {
data: {
userId,
addressId,
},
});
}
- 响应数据示例
{
"code": 0,
"msg": "删除成功",
"data": {
"userId": 1,
"addressId": 1,
"area": "陕西省/西安市/雁塔区",
"areaCode": "610113",
"detailsAddress": "电子城街道科技园1栋5楼1206",
"receiver": "清心",
"phone": "12332222221",
"isDefault": false
}
}
大厂最新技术学习分享群
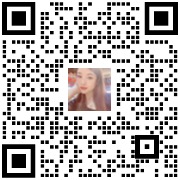
微信扫一扫进群,获取资料
X